For the past few posts, the main focus has been a NuSOAP Webservice that does really basic things with very limited data. The different functions of the service have done a variety of things:
- showNumbers – Returns an array of numbers in a non-assoc array key format
- showMadLib – Returns a mad lib based on the data it is sent. Need 4 Adjectives, 2 Adverbs, 3 Nouns, 1 Verb, and 2 Verb: Past Tense. It replies with a string that contains the madlib.
- showGroupItems – Simplistic service to return an array of items for a specific event, the ones available are: movie, picnic, drive, shopping
- showTaxes – Shows the taxes based on state taxes. Currently, the only states available in this are: AZ,AL,AK, CA, OR, WA, UT, ID, WY, Defaults to CA if no state is provided. The input for this function is an array.
- showPhrases – Shows a phrase from Shakespeare and replaces the name with the supplied name. ID is a number from 0 – 4
Although these are very basic and the real world applications are very limited, it can provide a spring board to building real world webservices that can be used for a variety of reasons. But, the power of webservices are that they can be code independent if written correctly. The server portion we have done is all in PHP. The client we have tested this on has been a PHP base. Based on the client functioning properly, we know that PHP based applications will be able to access the data. But the web is not 100% PHP based. Other code exists, which could be .NET flavors, Java, even ColdFusion. We need to ensure that the webservice built will be accepted by applications written in other languages.
This is why it is important to do cross platform/cross code clients for testing. It does not need to be a huge task or a full app. What I do is test it in the .NET C# command line. First, you will need to get some kind of Visual Studio if you do not already have it. I am not saying you have to spend thousands on Visual Studio, as they have great free IDEs available. The one I use is Visual Studio Express C# edition 2008. This is a FREE download, and can be located at
http://www.microsoft.com/express/Downloads/#2008-Visual-CS
Once that is installed you are ready to go. The first thing to do is to create a new project. I select a command line project as that will spit out data and let me know it was capable of getting the data. I created my project under the name of “HirdWebClient”, then clicked OK.
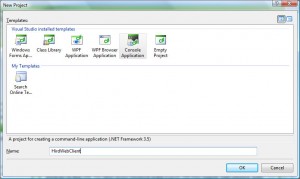
After it creates the project, there are a couple if items to include. Click on the “Reference” folder and add the following references if they are not already there. (I may be including more than needed, and that is ok, we are not packaging this to anyone and just testing)
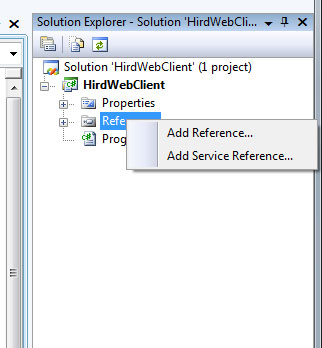
Add the following references:
System.Web.Services
System.ServiceModel
System.Xml
System.Collections
Make sure to add these to the top of the code as well. So the header on the “Program.cs” should look like:
using System; using System.Collections.Generic; using System.Web; using System.Web.Services; using System.Linq; using System.Text; using System.ServiceModel; using System.Xml; using System.Collections;
Next, click the “References” folder again and select “Add Service Reference”. This will open a new window with a place to enter the WSDL address and to name the service (if you do not want the default).
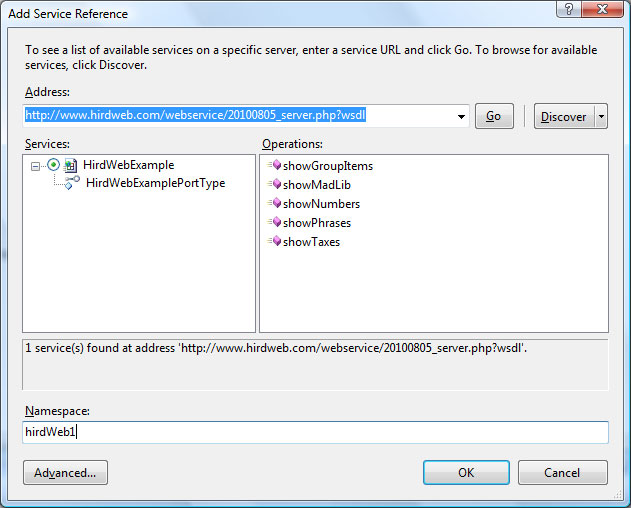
In this example, I added the WSDL address:
http://www.hirdweb.com/webservice/20100805_server.php?wsdl
After clicking “go”, I named the Namespace “hirdWeb1”. In the example, you can see that all the functions are found. So far, so good. Click “OK”. It takes me back to the Program.cs file, and now I can “Build”, “Build Solution”, or just press F6. This will save the project (if you have not already done so), and check for any errors. You should not receive any errors as there is really no code yet.
The Code should look like this to start:
namespace HirdWebClient { class Program { static void Main(string[] args) { } } }
In the “static void Main(string[] args)” function we will be placing all the code. In this post, I will only be checking three functions: showPhrases, showNumbers, and showTaxes. First we will start with the showPhrases. This just takes two input values, an ID and a name. But, we should declare the webservice first. In .NET, the way that the service is defined is by using a “PortTypeClient”. I renamed the webservice to erase all spaces to: HirdWebExample
$server->configureWSDL('HirdWebExample','HirdWeb Basic Webservice Example', $wsdl_addr);
So to get this webservice going and ready for use, we need to declare it as a n object and name the object, and define it as a new PortTypeClient object. Then, for ease of use, declare two variables for ID and NAME.
namespace HirdWebClient { class Program { static void Main(string[] args) { hirdWeb1.HirdWebExamplePortTypeClient hw = new hirdWeb1.HirdWebExamplePortTypeClient(); string id = "0"; string uname = "Maurice Moss"; } } }
The good thing about the Visual Studio IDE is that helps those that may not be fluent in the language. It provides drop downs when you start typing to help guide you. Now we need to declare a result for the returning data, and call the function. Once the resultset has the data, we can print it out. Since it is a simple single string returned, it is easy to print out. And remember in C# the print statement is Console.Write or Console.WriteLine.
namespace HirdWebClient { class Program { static void Main(string[] args) { hirdWeb1.HirdWebExamplePortTypeClient hw = new hirdWeb1.HirdWebExamplePortTypeClient(); string id = "0"; string uname = "Maurice Moss"; hirdWeb1.showPhrases res1 = hw.showPhrases(id, uname); Console.WriteLine(res1.phrase); } } }
Now rebuild the application, then click on the menu, “Debug”, then “Start Without Debugging” (or Ctrl and F5). If there are no errors during build, you should get the following to show up:
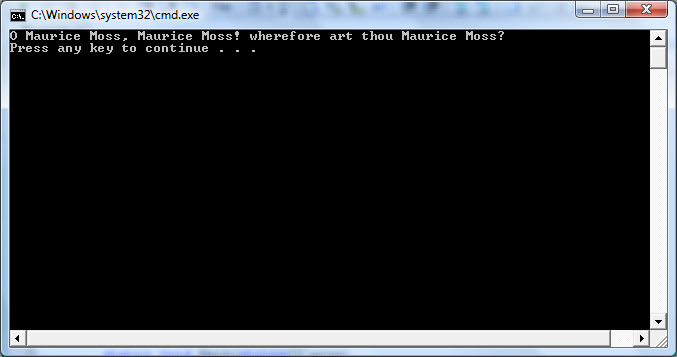
Now we need to add in the showNumbers, and then print it out. However, since it returns an array of “strings” (which are just numbers anyway), we can not just do a Console.WriteLine. We must create a string to print these to in a foreach loop. This can be done by adding the following lines of code to the above:
hirdWeb1.showNumbers res2 = hw.showNumbers(“4”);
foreach ( string num in res2.numbers){
Console.WriteLine(num);
}
Rebuild the solution, then Start Without Debugging, and you should get the following:
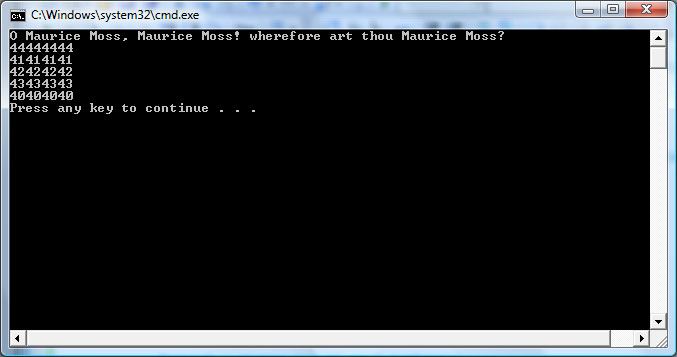
Whew. So now we know that the webservice is working and returning data to non-PHP applications. With one exception, the showTaxes requires an array of data. We must also ensure that the array of data is allowed to be passed in order to work. In C#, this is done a little differently.
In order to get the array to the webservice, we will not be building an array in the traditional sense, but creating an object that will add the correct data to the correct array element. First we need to instantiate the “inTaxArray” object that the webservice is going to need.
hirdWeb1.inTaxArray tax = new hirdWeb1.inTaxArray();
This is using the hirdWeb1 service reference, and creating a new “inTaxArray” object named “tax”. Now that the object is created, we need to add the correct items. This is done via the “Add” method. One element is “price” and the other is “state”. By using the Add method, we can assign those items values.
tax.price = "4.99"; tax.state = "CA";
We now have the array object ready to pass to the webservice.
hirdWeb1.showTaxes res3 = hw.showTaxes(tax);
Now that it is passed, we need to print it out, and make sure it is good. It returns an array in the “taxes” element. So we will need to reference that space when we do the print out of the data. And since the data may not be easily read as just data, we will also add some labels for the data that is being printed out.
Console.WriteLine("Original Price: " + res3.taxes.price); Console.WriteLine("Tax on the Price: " + res3.taxes.tax); Console.WriteLine("TOTAL AMOUNT: " + res3.taxes.total); Console.WriteLine("Tax State: " + res3.taxes.state);
In C#, to concatenate strings, use the “+” sign, instead of the “.” sign. This will print out the each item from the returned result. And since we now have three different functions, let’s also make it look a little easier to read. So we will add some separators to the printout. The full code of the Program.cs file is below:
using System; using System.Collections.Generic; using System.Web; using System.Web.Services; using System.Linq; using System.Text; using System.ServiceModel; using System.Xml; using System.Collections; namespace HirdWebClient { class Program { static void Main(string[] args) { hirdWeb1.HirdWebExamplePortTypeClient hw = new hirdWeb1.HirdWebExamplePortTypeClient(); // Create variables for the showPhrases function string id = "0"; string uname = "Maurice Moss"; // Create variables for the showTaxes input array hirdWeb1.inTaxArray tax = new hirdWeb1.inTaxArray(); tax.price = "4.99"; tax.state = "CA"; // Call each function to a resultset object hirdWeb1.showPhrases res1 = hw.showPhrases(id, uname); hirdWeb1.showNumbers res2 = hw.showNumbers("4"); hirdWeb1.showTaxes res3 = hw.showTaxes(tax); // Print out all the results Console.WriteLine(res1.phrase); Console.WriteLine("================================"); foreach ( string num in res2.numbers){ Console.WriteLine(num); } Console.WriteLine("================================"); Console.WriteLine("Original Price: " + res3.taxes.price); Console.WriteLine("Tax on the Price: " + res3.taxes.tax); Console.WriteLine("TOTAL AMOUNT: " + res3.taxes.total); Console.WriteLine("Tax State: " + res3.taxes.state); } } }
And that should now look like the following:
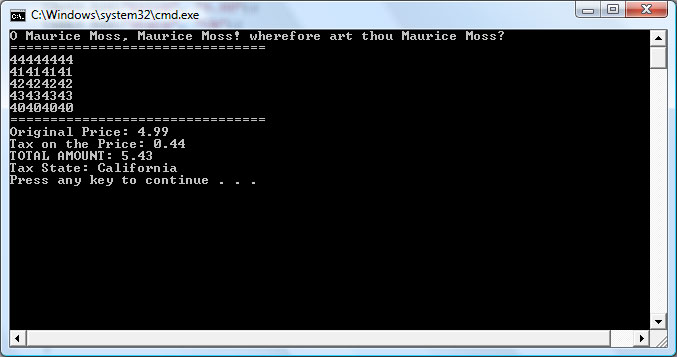
And that just about finishes up the whole NuSOAP experience. The C# command line application was able to query the PHP data and get the returned output and print it out. It now works well with PHP and C# and we have tested it in both languages. Now it is time to go out there and create and consume webservices.